Understanding environment variables in SvelteKit
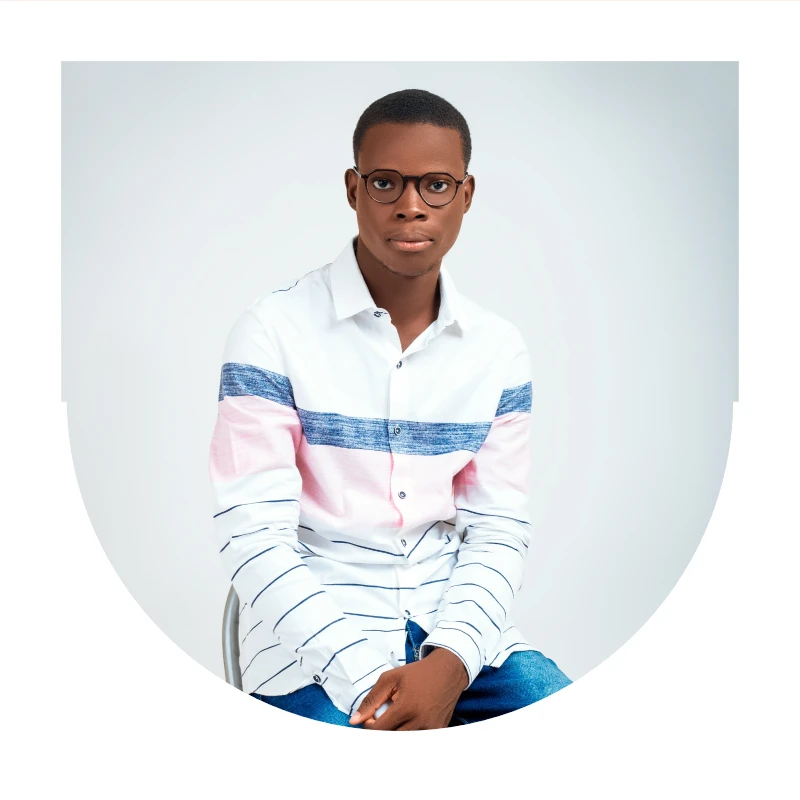
Justin Ahinon
Last updated on
SvelteKit version: 1.5.0
Environment variables are probably one of the most used features in NodeJS applications. They allow storing of sensitive information such as API keys, database credentials, and other information that should not be stored in the codebase.
SvelteKit has very great first-class support for environment variables. From type checking to server/client-side access, the meta-framework makes accessing and consuming environment variables very easy.
Accessing environment variables in a Node.js application
The most straightforward way to access environment variables in a Node.js application is to prefix the execution command with the environment variable. For example, to set the environment variable NODE_ENV
to production
, you can run the following command:
code loading...
assumes that the index.js file is the application's entry point.
This method can quickly become tedious to scale when you have multiple variables to set. That’s why Node.js provides the process.env
global variable that’s injected at runtime. process.env
can be used to access environment variables present on the system where the application is running and set in the application itself.
code loading...
code loading...
This will print the PATH
environment variable to the console.
Environment variables: the SvelteKit way
At its core, SvelteKit is a Vite plugin that runs the Svelte compiler. That means any SvelteKit application inherits the environment variables features that are provided by Vite.
You can then load and use in almost all the parts of your SvelteKit application the following environment variables:
import.meta.env.MODE
- The current mode of the application. It can be either development or production.import.meta.env.BASE_URL
- The base URL of the application.import.meta.env.PROD
- A boolean that indicates whether the application is running in production mode.import.meta.env.DEV
- A boolean that indicates whether the application is running in development mode.import.meta.env.SSR
- A boolean that indicates whether the application is running in server-side rendering mode.
Also, per default, only environment variables defined in the .env
file that starts with PUBLIC_
can be accessed by client-side code by running import.meta.env.PUBLIC_*
. All the other environment variables are only accessible by server-side code.
Note that the PUBLIC_
prefix is the default, and this configuration can be changed in the svelte.config.js
file.
Most of the time, you will not need to use the import.meta.env
global variable directly. This is because SvelteKit comes with some handy features that make it very seamless to use environment variables. With those features, you can access the variables in a type-safe way and clearly separate client and server-side variables. Let’s see how.
Private static environment variables
Static environment variables are loaded by Vite from the .env
file using process.env
. These variables are likely to be used by the server-side code. Think of database credentials, API keys, and other sensitive information that should not be stored in the codebase.
These variables are statically injected at build time in the code and must not be prefixed with the public prefix for client-side variables ( PUBLIC_
by default).
To load static environment variables, you can create a .env
file at the root of your project and add the variables you want to load. For example, to load the DATABASE_URL
environment variable, you can add the following line to the .env
file:
code loading...
code loading...
“Server-side code” is any code that runs in the +page.server.js
or +service.js
files.
Public static environment variables
Public static environment variables work almost the same way as private static environment variables. The only difference is that they are prefixed with the public prefix for client-side variables ( PUBLIC_
by default).
code loading...
code loading...
Private dynamic environment variables
These variables are accessed during runtime. They are dynamic because they can change at runtime and might vary depending on the environment where the application is running.
code loading...
Public dynamic environment variables
They work the same way as private dynamic environment variables but are prefixed with the public prefix for client-side variables ( PUBLIC_
by default).
The documentation for environment variables in SvelteKit can be found here:
TypeScript support
SvelteKit comes with great support for TypeScript. That means that you can use environment variables in a type-safe way. For example, if you have the following environment variable:
code loading...
And you try to call it in your code:
code loading...
you will get a type error because the APID_KEY
variable is not defined in the env.d.ts
file (note the “D” at the end of API). To fix this, you can add the following line to the .svelte-kit/ambient.d.ts
file. This provides a great developer experience and makes it easy to call environment variables properly.
More interestingly, you can not directly use variables imported from private static and private dynamic modules in Svelte components ( .svelte
files), which are client-side.
Conclusion
Environment variables are a great way to configure your application. They are easy to use and provide a great developer experience. SvelteKit makes it even easier to use them and provides great support for TypeScript.
Once you get to know how they work, you will wonder how you could have lived without them.
Get help with your Svelte or SvelteKit code
Got a Svelte/Sveltekit bug giving you headaches? I’ve been there!
Book a free 15 min consultation call, and I’ll review your code base and give you personalized feedback on your code.
P.S. If you have a bigger problem that may need more than 15 minutes, you can also pick my brain for a small fee. 😊 But that’s up to you!
Environment variables are sensitive information such as API keys and database credentials that should not be stored in the codebase. SvelteKit provides first-class support for environment variables.
You can prefix the execution command with the environment variable or use the process.env
global variable that's injected at runtime.
SvelteKit allows you to use variables like import.meta.env.MODE
, import.meta.env.BASE_URL
, import.meta.env.PROD
, import.meta.env.DEV
, and import.meta.env.SSR
.
SvelteKit allows access to environment variables starting with PUBLIC_
from the client-side code. All other environment variables are private and accessible only by server-side code.
Private static environment variables are loaded by Vite from the .env
file using process.env
and are primarily used by server-side code. These variables are injected at build time in the code and must not be prefixed with PUBLIC_
.
Public static environment variables function similarly to private static variables but are prefixed with PUBLIC_
for access by client-side code.
Dynamic environment variables are accessed during runtime and can change depending on the environment where the application is running. SvelteKit supports both private and public dynamic environment variables.
SvelteKit allows you to use environment variables in a type-safe way. You will get a type error if a variable is not defined correctly, thus enhancing the developer experience.
The overall developer experience with SvelteKit and TypeScript is absolutely great, and we definitely recommend using SvelteKit with TypeScript. Check out this article where we expand more about that: Why you should use TypeScript in your next SvelteKit projects.
No, you cannot directly use variables imported from private static and private dynamic modules in Svelte components, which are client-side.
Environment variables provide an easy and secure way to configure your application. With SvelteKit's robust support, they offer an enhanced developer experience.